Complete guide to Keywords in C Programming
- Technology addicts
- Jul 18, 2023
- 4 min read
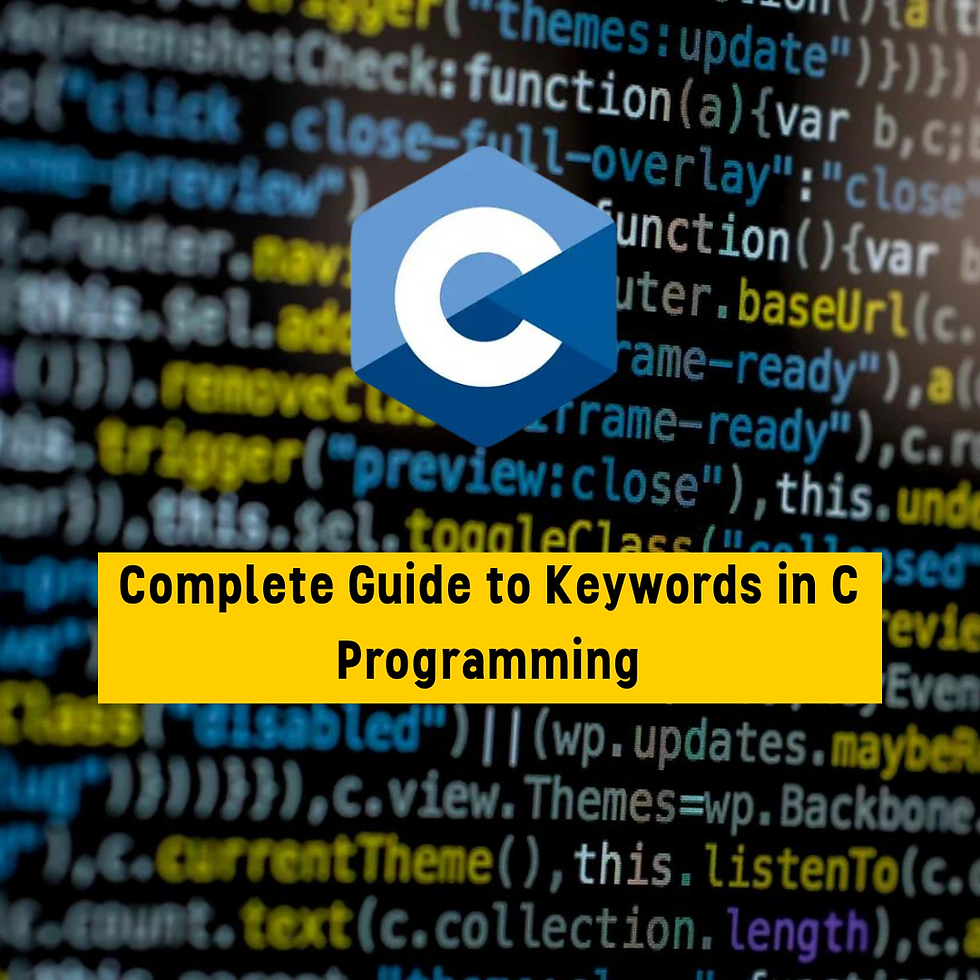
Keywords in C programming are reserved words that have predefined meanings and functionalities. These words cannot be used as variable names or identifiers because they are already used by the C language itself. Here's a complete guide to keywords in C programming:
1. Auto:
This keyword is used to declare automatic variables. It has limited use in modern C programming and is rarely used.
2. Break:
The "break" keyword is used in switch statements and loops (like "for," "while," and "do-while") to exit the loop or switch block prematurely.
3. Case:
The "case" keyword is used in switch statements to define specific cases or values to be compared with the switch expression.
4. Char:
"char" is a data type used to represent characters, such as letters and symbols.
5. Const:
The "const" keyword is used to declare constant variables whose values cannot be modified after initialization.
6. Continue:
The "continue" keyword is used in loops to skip the remaining iterations and jump to the next iteration.
7. Default:
The "default" keyword is used in switch statements to define the default case when none of the other cases match the switch expression.
8. Do:
The "do" keyword is used to start a "do-while" loop, which executes a block of code while a specified condition is true.
9. Double:
"double" is a data type used to store double-precision floating-point numbers with more precision than "float."
10. Else:
The "else" keyword is used in conditional statements (like "if" statements) to specify an alternative block of code to execute when the condition is false.
11. Enum:
The "enum" keyword is used to define a set of named constants representing integral values.
12. Extern:
The "extern" keyword is used to declare variables or functions that are defined in other source files.
13. Float:
"float" is a data type used to store single-precision floating-point numbers.
14. For:
The "for" keyword is used to start a "for" loop, which repeatedly executes a block of code based on a specified condition.
15. Goto:
The "goto" keyword is used to transfer the program's control to a labeled statement. Its use is discouraged in modern programming due to its potential to create unreadable and error-prone code.
16. If:
The "if" keyword is used to start a conditional statement that executes a block of code if a given condition is true.
17. Int:
"int" is a data type used to store integer values.
18. Long:
"long" is a data type used to store integer values with a larger range than "int."
19. Register:
The "register" keyword suggests that the variable should be stored in a register for faster access. However, its use is not widely supported in modern C programming.
20. Return:
The "return" keyword is used in functions to return a value to the calling function.
21. Short:
"short" is a data type used to store integer values with a smaller range than "int."
22. Signed:
The "signed" keyword is used to declare variables that can store both positive and negative integer values.
23. Sizeof:
The "sizeof" keyword is used to determine the size, in bytes, of a data type or a variable.
24. Static:
The "static" keyword is used to declare static variables, which retain their values between function calls.
25. Struct:
The "struct" keyword is used to define a user-defined data structure that can hold multiple variables of different data types.
26. Switch:
The "switch" keyword is used to start a switch statement, which provides a way to choose one of many blocks of code to be executed based on a single expression.
27. Typedef:
The "typedef" keyword is used to create new data type aliases, making code more readable and maintainable.
28. Union:
The "union" keyword is used to define a user-defined data type that can hold multiple variables of different data types but shares the same memory location.
29. Unsigned:
The "unsigned" keyword is used to declare variables that can store only positive integer values.
30. Void:
"void" is a data type used to indicate the absence of a specific type, typically used in function return types when a function does not return a value.
31. Volatile:
The "volatile" keyword is used to indicate that a variable's value can be changed by an external entity, and the compiler should not perform any optimization on it.
These are the keywords in the C programming language. It's essential to be familiar with them to write valid and correct C programs. Avoid using keywords as variable names to prevent conflicts and ensure smooth program execution.
In conclusion, keywords are reserved words in the C programming language that have predefined meanings and functionalities. They cannot be used as variable names or identifiers because they are already used by the language itself. Understanding keywords is essential for writing valid and effective C programs.
A comprehensive C tutorial will cover the basics of keywords, explaining their specific purposes and how to use them correctly. Learners will gain knowledge about data types (such as int, float, and char) and control flow keywords (like if, else, for, while, and switch) to make decisions and control program flow. Additionally, the tutorial will emphasize the significance of avoiding keyword usage as variable names to prevent errors.
By mastering keywords and basics in C, students can build a strong foundation for their programming journey and develop efficient, error-free C programs for various applications.
Comments