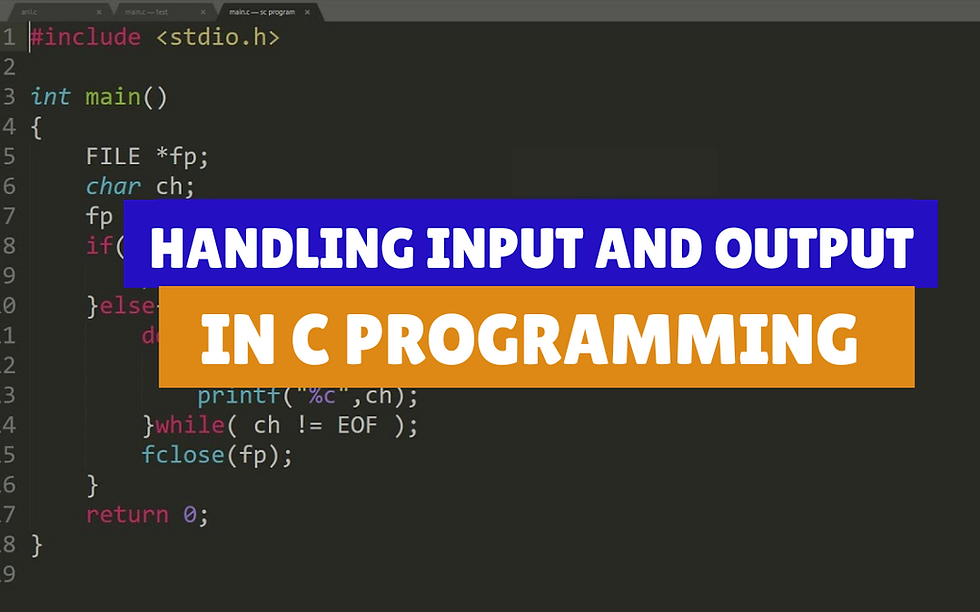
In C programming, input and output operations play a crucial role in interacting with users and managing data. Understanding how to handle input and output effectively is essential for building robust and functional applications. In this article, we will delve into various methods and techniques for handling input and output in C. We will explore file handling, which allows us to read from and write to files, as well as standard I/O functions, which enable communication with the user through the console. By mastering these concepts, you will be able to efficiently manage data, store information, and create interactive programs.
Learn from C tutorial will cover the intricacies of handling input and output operations in C. You will learn about standard I/O functions like `scanf()` and `printf()` for console-based communication, as well as file handling techniques using functions such as `fopen()`, `fclose()`, `fread()`, and `fwrite()`. These skills will enable you to interact with users, manage data stored in files, and create programs that efficiently process input and produce output.
1. Standard Input and Output
In C, standard input (stdin) and standard output (stdout) are the default sources and destinations of data respectively. They provide a convenient way to interact with the user through the console. The <stdio.h> header file provides several functions for handling standard I/O operations, such as `scanf()` for input and `printf()` for output. These functions allow you to format and display data, as well as read input from the user. You can use format specifiers to control the output formatting and extract data from the input stream using variables and conversion specifiers.
2. File Handling
File handling in C allows you to read data from and write data to external files. This is useful for tasks like reading input from a file, writing output to a file, or manipulating data stored in files. The <stdio.h> header file provides functions like `fopen()`, `fclose()`, `fread()`, and `fwrite()` for file operations. These functions allow you to open files, close files, read data from files, and write data to files, respectively. You can specify the file mode (e.g., read, write, append) when opening a file, and handle errors using return values and error codes.
3. Opening and Closing Files
Before performing any operations on a file, it needs to be opened using the `fopen()` function. This function takes the file name and mode as arguments and returns a file pointer that represents the opened file. The file mode can be "r" for reading, "w" for writing, or "a" for appending, among others. Once you are done with the file, it should be closed using the `fclose()` function. Closing the file is important to release system resources and ensure data integrity.
4. Reading from Files
To read data from a file, you can use the `fread()` function. This function takes several arguments, including a buffer to store the read data, the size of each element to be read, the number of elements to read, and the file pointer. It returns the number of elements successfully read. You can process the data read from the file as per your requirements. Error handling should be implemented to handle cases where the file is not available or the read operation fails.
5. Writing to Files
To write data to a file, you can use the `fwrite()` function. This function takes arguments similar to `fread()`, including a buffer containing the data to be written, the size of each element, the number of elements to write, and the file pointer. It returns the number of elements successfully written. You can format the data before writing it to the file using string formatting functions like `sprintf()` or by directly writing to the file using `fprintf()`. Proper error handling is necessary to handle write failures and ensure the integrity of the written data.
6. Error Handling
Error handling is an important aspect of input and output operations in C. It allows you to detect and handle errors that may occur during file handling or standard I/O operations. Functions like `feof()` and `ferror()` can be used to check for end-of-file conditions and file errors, respectively. Additionally, you can use `perror()` to display descriptive error messages corresponding to specific error codes. Proper error handling ensures that your program gracefully handles exceptional situations and provides useful feedback to the user.
7. File Positioning
File positioning allows you to move the file pointer within a file, enabling you to read or write data at specific locations. The `fseek()` function is used to move the file pointer to a specified position, based on a given offset and a reference point. The reference point can be the beginning of the file, the current position, or the end of the file. The `ftell()` function returns the current position of the file pointer. By manipulating the file position, you can read or write data at specific positions within a file, enabling tasks such as random access or updating specific records.
Also Read: Pointers in C Complete Guide
Conclusion
Handling input and output operations effectively is crucial for developing functional and interactive programs in C. File handling allows you to read from and write to external files, providing a means to store and retrieve data persistently. Standard I/O functions facilitate communication with the user through the console. By mastering these techniques, you can efficiently manage data, create interactive applications, and handle various scenarios such as error detection and file positioning. By implementing proper error handling, ensuring data integrity, and utilizing the power of file handling and standard I/O functions, you can build robust C programs that effectively handle input and output operations.
In conclusion, learning the basics of C programming and understanding how to handle input and output operations are fundamental skills for any aspiring programmer. By following a comprehensive C language tutorial, you can gain a solid foundation in the language and develop proficiency in handling input and output effectively.
A C language tutorial will introduce you to the core concepts of C programming, including variables, data types, control structures, functions, and arrays. It will provide you with the knowledge to write clean and structured code, ensuring proper syntax and logical flow.
By mastering these concepts through a C language tutorial, you will gain the necessary skills to tackle programming challenges, develop practical applications, and lay the foundation for further advancements in the field of computer programming.
Comentários