Data Types and Variables in C Programming Language
- Technology addicts
- Jul 31, 2023
- 4 min read
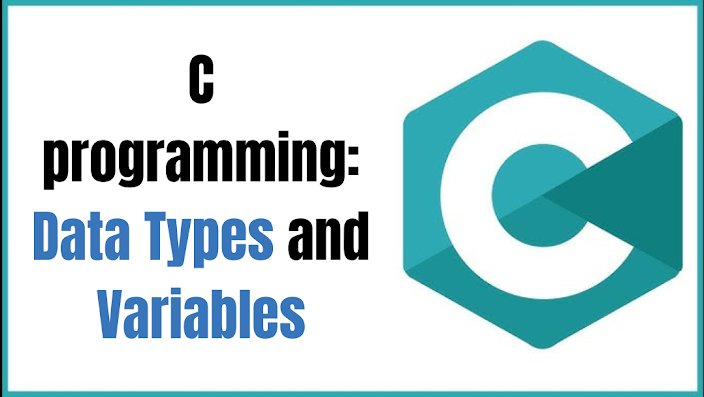
In C programming, data types and variables are essential concepts used to store and manipulate different types of data. Understanding data types and variables is crucial for effective programming. This tutorial will explain the various data types available in C and how to declare and use variables.
Data Types in C:
C provides several data types that define the size and behavior of variables. Here are the commonly used data types in C:
1. Integer Types:
- `int`: The `int` data type represents integers, which are whole numbers (both positive and negative) without fractional parts. It typically uses 4 bytes of memory.
- `short`: The `short` data type represents integers with a smaller range than `int`. It usually uses 2 bytes of memory.
- `long`: The `long` data type represents integers with a larger range than `int`. It typically uses 4 or 8 bytes of memory, depending on the system.
2. Floating-Point Types:
- `float`: The `float` data type represents single-precision floating-point numbers. It is used to store numbers with decimal places and can typically store up to 6-7 significant digits.
- `double`: The `double` data type represents double-precision floating-point numbers. It can store more significant digits than `float` and is commonly used for higher precision calculations.
3. Character Types:
- `char`: The `char` data type is used to store single characters. It typically uses 1 byte of memory and can represent both printable characters and special control characters.
4. Derived Types:
- `array`: An array is a collection of elements of the same data type. It allows storing multiple values under a single variable name.
- `pointer`: A pointer is a variable that stores the memory address of another variable. It is used for dynamic memory allocation and accessing data indirectly.
- `structure`: A structure is a user-defined data type that allows combining multiple variables of different types into a single entity.
- `union`: A union is a data type that enables storing different types of data in the same memory space.
Variable Declaration and Initialization:
To use a variable in C, it must be declared with its appropriate data type. Variable declaration involves specifying the variable name and its data type. Here's the general syntax for variable declaration:
data_type variable_name;
For example:
int age;
float pi;
char grade;
Variables can also be initialized during declaration by assigning an initial value. Here's an example:
int age = 25;
float pi = 3.14;
char grade = 'A';
Variable initialization is optional, and variables can be assigned values later in the program.
Variable Assignment and Usage:
Variables in C are used to store and manipulate data. They can be assigned new values and used in expressions. Here are some examples:
int a = 10;
int b = 20;
int sum = a + b; // Adding two variables and storing the result in another variable
printf("The sum is: %d\n", sum); // Printing the value of the sum variable
In the above code, two variables `a` and `b` are declared and assigned values. The sum of `a` and `b` is calculated and stored in the `sum` variable. Finally, the value of `sum` is printed using `printf()`.
Variable Scope and Lifetime:
Variables in C have a scope and lifetime that determine their visibility and availability in different parts of the program. Here are the main aspects of variable scope and lifetime:
1. Scope:
- Global Scope: Variables declared outside of any function have global scope and can be accessed from any part of the program.
- Local Scope: Variables declared inside a function have local scope and can only be accessed within that function.
2. Lifetime:
- Automatic Variables: Variables declared inside a function without the `static` keyword have automatic storage duration. They are created when the function is called and destroyed when the function completes.
- Static Variables: Variables declared inside a function with the `static` keyword have static storage duration. They retain their values between function calls.
Type Modifiers and Qualifiers:
C provides modifiers and qualifiers to modify the behavior and properties of data types. Here are a few commonly used modifiers and qualifiers:
1. `signed` and `unsigned`: These modifiers are used with integer types to specify whether the values can be negative or non-negative.
2. `const`: The `const` qualifier is used to define constants, i.e., variables whose values cannot be changed once assigned.
3. `volatile`: The `volatile` qualifier is used to indicate that a variable can be modified by external entities, such as hardware devices.
4. `typedef`: The `typedef` keyword is used to create aliases for existing data types, allowing the programmer to define custom names for them.
Type Conversion and Casting:
C supports implicit and explicit type conversions. Implicit conversion occurs automatically when assigning values of one type to another compatible type. Explicit type conversion, also known as casting, is done explicitly by the programmer. Here's an example of typecasting:
int a = 10;
double b = (double)a; // Casting 'a' to double type
In the above code, the variable `a` is explicitly cast to a `double` type using `(double)a`.
Conclusion:
Data types and variables are fundamental concepts in C programming. Understanding the available data types, declaring and initializing variables, and manipulating them are crucial for writing effective and efficient C programs. By mastering data types and variables, you can handle various types of data and perform complex computations in your C programs. Continually practicing and exploring these concepts will help you become proficient in C programming and enable you to solve a wide range of programming problems.
In conclusion, this C tutorial has provided an overview of data types and variables, which are fundamental concepts in programming. We covered the different data types available in C, including integers, floating-point numbers, characters, and derived types like arrays and structures. We also learned how to declare and initialize variables, as well as perform operations using them.
Understanding data types and variables allows us to store and manipulate data effectively in our programs. By mastering these concepts, we can write more complex programs, handle different types of data, and solve a wide range of programming problems.
To further enhance your C programming skills, it is recommended to practice implementing programs that involve various data types and variables. Additionally, exploring advanced topics like type modifiers, type conversions, and memory management will deepen your understanding of C programming. Continued practice and exploration will contribute to becoming a proficient C programmer.
Σχόλια